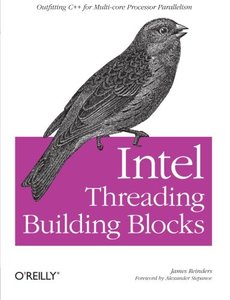
Intel Threading Building Blocks: Outfitting C++ for Multi-core Processor Parallelism (Paperback)
內容描述
Description
Multi-core chips from Intel and AMD offer a
dramatic boost in speed and responsiveness, and plenty of opportunities for
multiprocessing on ordinary desktop computers. But they also present a
challenge: More than ever, multithreading is a requirement for good
performance. This guide explains how to maximize the benefits of these
processors through a portable C++ library that works on Windows, Linux,
Macintosh, and Unix systems. With it, you'll learn how to use Intel Threading
Building Blocks (TBB) effectively for parallel programming -- without having
to be a threading expert.Written by James Reinders, Chief Evangelist
of Intel Software Products, and based on the experience of Intel's developers
and customers, this book explains the key tasks in multithreading and how to
accomplish them with TBB in a portable and robust manner. With plenty of
examples and full reference material, the book lays out common patterns of
uses, reveals the gotchas in TBB, and gives important guidelines for choosing
among alternatives in order to get the best performance.You'll learn
how Intel Threading Building Blocks:
Enables you to specify tasks instead of threads for better
portability, easier programming, more understandable source code, and better
performance and scalability in general
Focuses on the goal of parallelizing computationally intensive work
to deliver high-level solutions
Is compatible with other threading packages, and doesn't force you
to pick one package for your entire program
Emphasizes scalable, data-parallel programming, which allows program
performance to increase as you add processors
Relies on generic programming, which enables you to write the best
possible algorithms with the fewest constraints Any C++
programmer who wants to write an application to run on a multi-core system
will benefit from this book. TBB is also very approachable for a C programmer
or a C++ programmer without much experience with templates. Best of all, you
don't need experience with parallel programming or multi-core processors to
use this book.
Table of Contents
Foreword
Note from the Lead Developer of Intel Threading Building Blocks
Preface
- Why Threading Building Blocks?
Overview Benefits Thinking Parallel Elements of
Thinking Parallel Decomposition
Scaling and Speedup What Is a
Thread? Mutual Exclusion and Locks
Correctness Abstraction
Patterns IntuitionBasic Algorithms Initializing and
Terminating the Library Loop Parallelization
Recursive Range Specifications
Summary of Loops- Advanced Algorithms Parallel
Algorithms for Streams - Containers concurrent_queue
concurrent_vector
concurrent_hash_map - Scalable Memory Allocation
Limitations Problems in Memory Allocation
Memory Allocators
Replacing malloc, new, and delete - Mutual Exclusion When to Use Mutual
Exclusion Mutexes
Mutexes Atomic Operations - Timing
- Task Scheduler When Task-Based
Programming Is Inappropriate Much Better Than Raw
Native Threads Initializing the Library Is Your
Job Example Program for Fibonacci Numbers
Task Scheduling Overview
How Task Scheduling Works Recommended Task
Recurrence Patterns Making Best Use of the
Scheduler Task Scheduler Interfaces
Task Scheduler Summary - Keys to Success Key Steps to Success
Relaxed Sequential Execution
Safe Concurrency for Methods and Libraries
Debug Versus Release For Efficiency's Sake
Enabling Debugging Features
Mixing with Other Threading Packages
Naming Conventions - Examples The Aha! Factor
A Few Other Key Points
parallel_for Examples The Game of Life
Parallel_reduce Examples
CountStrings: Using concurrent_hash_map
Quicksort: Visualizing Task Stealing A
Better Matrix Multiply (Strassen) Advanced Task
Programming Packet Processing Pipeline
Memory Allocation Game
Threading Example Physics Interaction and Update
Code Open Dynamics Engine - History and Related Projects
Libraries Languages
Pragmas Generic Programming
Caches Costs of Time Slicing
Quick Introduction to Lambda Functions
Further Reading
Index